[acool@localhost websocket-fun]$
[acool@localhost websocket-fun]$ # start web server
[acool@localhost websocket-fun]$ php -S localhost:8080
PHP 5.6.29 Development Server started at Fri Jul 28 22:21:15 2017
Listening on http://localhost:8080
Document root is /home/acool/websocket-fun
Press Ctrl-C to quit.
[acool@localhost websocket-fun]$
[acool@localhost websocket-fun]$ # start websocket application
[acool@localhost websocket-fun]$ php -q server.php
Open two browser windows go to localhost:8080 and start chatting with yourself hehe
Window 1
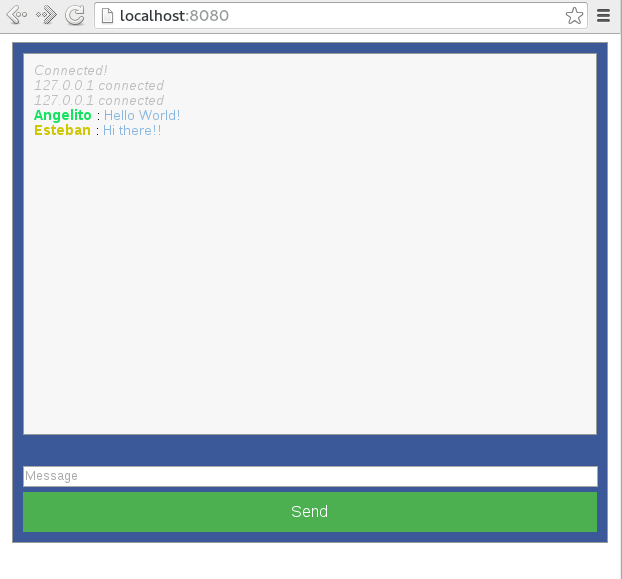
Window 2
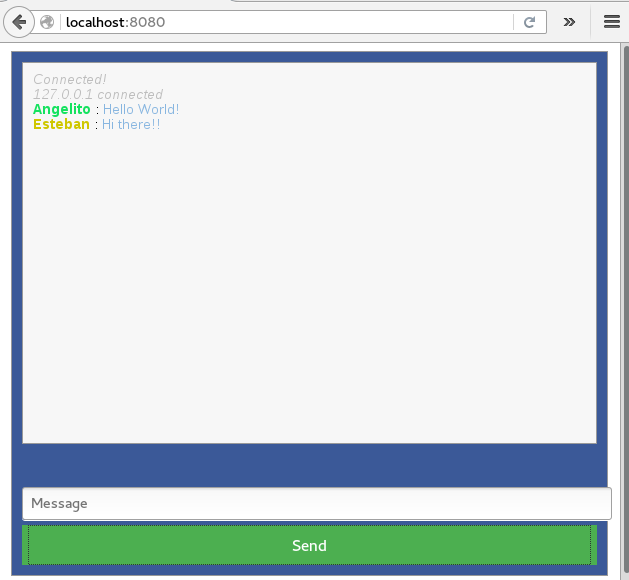
server.php
<?php
$host = 'localhost'; //host
$port = '9000'; //port
$null = NULL; //null var
//Create TCP/IP sream socket
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
//reuseable port
socket_set_option($socket, SOL_SOCKET, SO_REUSEADDR, 1);
//bind socket to specified host
socket_bind($socket, 0, $port);
//listen to port
socket_listen($socket);
//create & add listning socket to the list
$clients = array($socket);
//start endless loop, so that our script doesn't stop
while (true)
{
//manage multipal connections
$changed = $clients;
//returns the socket resources in $changed array
socket_select($changed, $null, $null, 0, 10);
//check for new socket
if (in_array($socket, $changed))
{
$socket_new = socket_accept($socket); //accpet new socket
$clients[] = $socket_new; //add socket to client array
$header = socket_read($socket_new, 1024); //read data sent by the socket
perform_handshaking($header, $socket_new, $host, $port); //perform websocket handshake
socket_getpeername($socket_new, $ip); //get ip address of connected socket
$response = mask(json_encode(array('type'=>'system', 'message'=>$ip.' connected'))); //prepare json data
send_message($response); //notify all users about new connection
//make room for new socket
$found_socket = array_search($socket, $changed);
unset($changed[$found_socket]);
}
//loop through all connected sockets
foreach ($changed as $changed_socket)
{
//check for any incomming data
while(socket_recv($changed_socket, $buf, 1024, 0) >= 1)
{
$received_text = unmask($buf); //unmask data
$tst_msg = json_decode($received_text); //json decode
$user_name = $tst_msg->name; //sender name
$user_message = $tst_msg->message; //message text
$user_color = $tst_msg->color; //color
//prepare data to be sent to client
$response_text = mask(json_encode(array('type'=>'usermsg', 'name'=>$user_name, 'message'=>$user_message, 'color'=>$user_color)));
send_message($response_text); //send data
break 2; //exist this loop
}
$buf = @socket_read($changed_socket, 1024, PHP_NORMAL_READ);
if ($buf === false)
{ // check disconnected client
// remove client for $clients array
$found_socket = array_search($changed_socket, $clients);
socket_getpeername($changed_socket, $ip);
unset($clients[$found_socket]);
//notify all users about disconnected connection
$response = mask(json_encode(array('type'=>'system', 'message'=>$ip.' disconnected')));
send_message($response);
}
}
}
// close the listening socket
socket_close($socket);
function send_message($msg)
{
global $clients;
foreach($clients as $changed_socket)
{
@socket_write($changed_socket,$msg,strlen($msg));
}
return true;
}
//Unmask incoming framed message
function unmask($text)
{
$length = ord($text[1]) & 127;
if($length == 126) {
$masks = substr($text, 4, 4);
$data = substr($text, 8);
}
elseif($length == 127) {
$masks = substr($text, 10, 4);
$data = substr($text, 14);
}
else {
$masks = substr($text, 2, 4);
$data = substr($text, 6);
}
$text = "";
for ($i = 0; $i < strlen($data); ++$i) {
$text .= $data[$i] ^ $masks[$i%4];
}
return $text;
}
//Encode message for transfer to client.
function mask($text)
{
$b1 = 0x80 | (0x1 & 0x0f);
$length = strlen($text);
if($length <= 125)
$header = pack('CC', $b1, $length);
elseif($length > 125 && $length < 65536)
$header = pack('CCn', $b1, 126, $length);
elseif($length >= 65536)
$header = pack('CCNN', $b1, 127, $length);
return $header.$text;
}
//handshake new client.
function perform_handshaking($receved_header,$client_conn, $host, $port)
{
$headers = array();
$lines = preg_split("/\r\n/", $receved_header);
foreach($lines as $line)
{
$line = chop($line);
if(preg_match('/\A(\S+): (.*)\z/', $line, $matches))
{
$headers[$matches[1]] = $matches[2];
}
}
$secKey = $headers['Sec-WebSocket-Key'];
$secAccept = base64_encode(pack('H*', sha1($secKey . '258EAFA5-E914-47DA-95CA-C5AB0DC85B11')));
//hand shaking header
$upgrade = "HTTP/1.1 101 Web Socket Protocol Handshake\r\n" .
"Upgrade: websocket\r\n" .
"Connection: Upgrade\r\n" .
"WebSocket-Origin: $host\r\n" .
// "WebSocket-Location: ws://$host:$port/demo/shout.php\r\n".
"Sec-WebSocket-Accept:$secAccept\r\n\r\n";
socket_write($client_conn,$upgrade,strlen($upgrade));
}
index.php
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<style type="text/css">
.panel{
margin-right: 3px;
}
.button {
background-color: #4CAF50;
border: none;
color: white;
margin-right: 30%;
margin-left: 30%;
text-decoration: none;
display: block;
font-size: 16px;
cursor: pointer;
width:30%;
height:40px;
margin-top: 5px;
}
input[type=text]{
width:100%;
margin-top:5px;
}
.chat_wrapper {
width: 70%;
height:472px;
margin-right: auto;
margin-left: auto;
background: #3B5998;
border: 1px solid #999999;
padding: 10px;
font: 14px 'lucida grande',tahoma,verdana,arial,sans-serif;
}
.chat_wrapper .message_box {
background: #F7F7F7;
height:350px;
overflow: auto;
padding: 10px 10px 20px 10px;
border: 1px solid #999999;
}
.chat_wrapper input{
//padding: 2px 2px 2px 5px;
}
.system_msg{color: #BDBDBD;font-style: italic;}
.user_name{font-weight:bold;}
.user_message{color: #88B6E0;}
@media only screen and (max-width: 720px) {
/* For mobile phones: */
.chat_wrapper {
width: 95%;
height: 40%;
}
.button{ width:100%;
margin-right:auto;
margin-left:auto;
height:40px;}
}
</style>
</head>
<body>
<?php
$colours = array('007AFF','FF7000','FF7000','15E25F','CFC700','CFC700','CF1100','CF00BE','F00');
$user_colour = array_rand($colours);
?>
<script src="jquery-3.1.1.js"></script>
<script language="javascript" type="text/javascript">
$(document).ready(function(){
//create a new WebSocket object.
var wsUri = "ws://localhost:9000/demo/server.php";
websocket = new WebSocket(wsUri);
websocket.onopen = function(ev) { // connection is open
$('#message_box').append("<div class=\"system_msg\">Connected!</div>"); //notify user
}
$('#send-btn').click(function(){ //use clicks message send button
var mymessage = $('#message').val(); //get message text
var myname = $('#name').val(); //get user name
if(myname == ""){ //empty name?
alert("Enter your Name please!");
return;
}
if(mymessage == ""){ //emtpy message?
alert("Enter Some message Please!");
return;
}
document.getElementById("name").style.visibility = "hidden";
var objDiv = document.getElementById("message_box");
objDiv.scrollTop = objDiv.scrollHeight;
//prepare json data
var msg = {
message: mymessage,
name: myname,
color : '<?php echo $colours[$user_colour]; ?>'
};
//convert and send data to server
websocket.send(JSON.stringify(msg));
});
//#### Message received from server?
websocket.onmessage = function(ev) {
var msg = JSON.parse(ev.data); //PHP sends Json data
var type = msg.type; //message type
var umsg = msg.message; //message text
var uname = msg.name; //user name
var ucolor = msg.color; //color
if(type == 'usermsg')
{
$('#message_box').append("<div><span class=\"user_name\" style=\"color:#"+ucolor+"\">"+uname+"</span> : <span class=\"user_message\">"+umsg+"</span></div>");
}
if(type == 'system')
{
$('#message_box').append("<div class=\"system_msg\">"+umsg+"</div>");
}
$('#message').val(''); //reset text
var objDiv = document.getElementById("message_box");
objDiv.scrollTop = objDiv.scrollHeight;
};
websocket.onerror = function(ev){$('#message_box').append("<div class=\"system_error\">Error Occurred - "+ev.data+"</div>");};
websocket.onclose = function(ev){$('#message_box').append("<div class=\"system_msg\">Connection Closed</div>");};
});
</script>
<div class="chat_wrapper">
<div class="message_box" id="message_box"></div>
<div class="panel">
<input type="text" name="name" id="name" placeholder="Your Name" maxlength="15" />
<input type="text" name="message" id="message" placeholder="Message" maxlength="80"
onkeydown = "if (event.keyCode == 13)document.getElementById('send-btn').click()" />
</div>
<button id="send-btn" class=button>Send</button>
</div>
</body>
</html>
Thanks to sanwebe.com
[ view entry ] ( 1504 views ) | print article
<?php
function isPalindrome($string=null)
{
if($string === null)
return 0;
if(is_numeric($string))
return 0;
$string = preg_replace('/\s+/', '', $string);
$reverse = strrev($string);
if($reverse == $string)
return 1;
else
return 0;
}
echo isPalindrome('lion oil');
[ view entry ] ( 1460 views ) | print article
Useful when having to insert a large set of data in chunks to a databse:
<?php
$numbers=array (
'1',
'2',
'3',
'4',
'5',
'6',
'7',
'8',
'9',
'10',
'11',
);
$output = array();
foreach($numbers as $i=>$number)
{
$output[]= $number;
if(($i+1) % 3 == 0 || $i==count($numbers)-1)//last key
{
var_dump($output);
$output=array();
}
}
The above outputs:
array(3) {
[0]=>
string(1) "1"
[1]=>
string(1) "2"
[2]=>
string(1) "3"
}
array(3) {
[0]=>
string(1) "4"
[1]=>
string(1) "5"
[2]=>
string(1) "6"
}
array(3) {
[0]=>
string(1) "7"
[1]=>
string(1) "8"
[2]=>
string(1) "9"
}
array(2) {
[0]=>
string(2) "10"
[1]=>
string(2) "11"
}
http://stackoverflow.com/questions/3549 ... -iteration
Thank you very much for your time !!!!!!
[ view entry ] ( 1557 views ) | print article
Amazing trick. This is one of the first thing to try if your CSV spreadsheet is not rendering how you expect.
Original Question:
======================================================
I have a line like this in my CSV:
"Samsung U600 24"","10000003409","1","10000003427"
Quote next to 24 is used to express inches, while the quote just next to that quote closes the field. I'm reading the line with fgetcsv but the parser makes a mistake and reads the value as:
Samsung U600 24",10000003409"
I tried putting a backslash before the inches quote, but then I just get a backslash in the name:
Samsung U600 24\"
Is there a way to properly escape this in the CSV, so that the value would be Samsung U600 24" , or do I have to regex it in the processor?
======================================================
Answer:
======================================================
Use 2 quotes:
"Samsung U600 24"""
======================================================
http://stackoverflow.com/questions/1780 ... ote-in-csv
[ view entry ] ( 1706 views ) | print article
/*********************************************************
|
| Creates Ajax-like request
|
*********************************************************/
function sendRequest($params,$url)
{
$postdata = http_build_query($params);
$opts = array('http' =>
array(
'method' => 'POST',
'header' => "Content-type: application/x-www-form-urlencoded \r\n".
"X-Requested-With: XMLHttpRequest \r\n",
'content' => $postdata
)
);
$context = stream_context_create($opts);
return json_decode(
file_get_contents($url.time(), false, $context)
);
}
$params=array(
'email' => 'me[at]example.com',
'choCountry' => 'Mexico',
'zip' => '91744',
);
$url= 'http://www.barney-example.com/subscribe';
$data = sendRequest($params,$url);
[ view entry ] ( 1786 views ) | print article
//SELECT * FROM tbl LIMIT 5,10; # Retrieve rows 6-15
$input = array("1","2","3","4","5","6","7","8","9","10","11","12","13","14","15","16","17","18","19");
print_r(array_slice($input,5,10));
Output:
Array
(
[0] => 6
[1] => 7
[2] => 8
[3] => 9
[4] => 10
[5] => 11
[6] => 12
[7] => 13
[8] => 14
[9] => 15
)
Complete Pagination Example:
function PaginateArray($input, $page, $show_per_page) {
$page = $page < 1 ? 1 : $page;
$start = ($page - 1) * ($show_per_page);
$offset = $show_per_page;
$outArray = array_slice($input, $start, $offset);
var_export($outArray);
}
$input = array("1","2","3","4","5","6","7","8","9","10","11","12","13","14","15","16","17","18","19","20");
//page 1
PaginateArray($input, 1, 3);//inputs: array, page, records per page
/*
array (
0 => '1',
1 => '2',
2 => '3',
)
*/
//page 2
PaginateArray($input, 2, 3);//inputs: array, page, records per page
/*
array (
0 => '4',
1 => '5',
2 => '6',
)
*/
[ view entry ] ( 1655 views ) | print article
//using table2csv plugin
//HTML:
<form action="getCSV.php" method ="post" >
<input type="hidden" name="csv_text" id="csv_text">
<input type="submit" value="Get CSV File"
onclick="getCSVData()"
</form>
<script>
function getCSVData(){
var csv_value=$('#tableID').table2CSV({delivery:'value'});
$("#csv_text").val(csv_value);
}
</script>
//php
<?php
header("Content-type: application/octet-stream");
header("Content-Disposition: attachment; filename=\"my-data.csv\"");
$data=stripcslashes($_REQUEST['csv_text']);
echo $data;
?>
[ view entry ] ( 1466 views ) | print article
Tested solution:
function CurrencyFormat($cash) {
// strip any commas
$cash = (0 + str_replace(',', '', $cash));
// filter and format it
if($cash>1000000000000){
return "$".round(($cash/1000000000000),1).'T';
}elseif($cash>1000000000){
return "$".round(($cash/1000000000),1).'B';
}elseif($cash>1000000){
return "$".round(($cash/1000000),1).'M';
}elseif($cash>1000){
return "$".round(($cash/1000),1).'K';
}
}
echo CurrencyFormat('1,560,000');//outputs $1.6M
[ view entry ] ( 2012 views ) | print article
//draft
$csv='...CSV file (string) exported from open office spread sheet';
$lines = explode("\n", $csv);
$array = array();
foreach ($lines as $line) {
$array =str_getcsv($line);
echo "INSERT INTO TestTable SET `Field1`='".addslashes($array[3])."',
`Field2`='".addslashes($array[3])."',";
}
[ view entry ] ( 1571 views ) | print article
<?php
/*
Sample data.
*/
$items = array(
array('id'=>1, 'title'=>'Home', 'parent_id'=>0),
array('id'=>2, 'title'=>'News', 'parent_id'=>1),
array('id'=>3, 'title'=>'Sub News', 'parent_id'=>2),
array('id'=>4, 'title'=>'Articles', 'parent_id'=>0),
array('id'=>5, 'title'=>'Article', 'parent_id'=>4),
array('id'=>6, 'title'=>'Article2', 'parent_id'=>4)
);
/*
Group by parent.
*/
$itemsByParent = array();
foreach ($items as $item)
{
if (!isset($itemsByParent[$item['parent_id']]))
$itemsByParent[$item['parent_id']] = array();
$itemsByParent[$item['parent_id']][] = $item;
}
/*
Print list recursively.
*/
function printList($items, $parentId = 0)
{
echo '<ul>';
foreach ($items[$parentId] as $item)
{
echo '<li>';
echo $item['title'];
$curId = $item['id'];
//if there are children
if (!empty($items[$curId]))
{
printList($items, $curId);
}
echo '</li>';
}
echo '</ul>';
}
/*
Finds top parent given node id
*/
function findTopParent($id,$ibp)
{
foreach($ibp as $parentID=>$children)
{
foreach($children as $child)
{
if($child['id']==$id)
{
if($child['parent_id']!=0)
return findTopParent($child['parent_id'],$ibp);
else
return $child;
}
}
}
}
/*
Get all parents, aka. breadcrumbs.
*/
function getAllParents($id,$ibp)
{
foreach($ibp as $parentID=>$nodes)
{
foreach($nodes as $node)
{
if($node['id']==$id)
{
if($node['parent_id']!=0)
{
$a=getAllParents($node['parent_id'],$ibp);
array_push($a,$node['parent_id']);
return $a;
}
else
return array();
}
}
}
}
/*
Gets all subnodes; children, grand children, etc...
*/
function getDescendants($id,$ibp)
{
if(array_key_exists($id,$ibp))
{
$kids=array();
foreach($ibp[$id] as $child)
{
array_push($kids,$child['id']);
if(array_key_exists($child['id'],$ibp))
$kids=array_merge($kids,getDescendants($child['id'],$ibp));
}
return $kids;
}
else
return array();//supplied $id has no kids
}
// Print it!!
printList($itemsByParent);
// Find top parent!!
print_r(findTopParent(6,$itemsByParent));
/*
Array
(
[id] => 4
[title] => Articles
[parent_id] => 0
)
*/
// Find path!!
print_r(getAllParents(3,$itemsByParent));
/*
Array
(
[0] => 1
[1] => 2
)
*/
print_r(getDescendants(1,$itemsByParent));
/*
Array
(
[0] => 2
[1] => 3
)
*/
print_r(getDescendants(4,$itemsByParent));
/*
Array
(
[0] => 5
[1] => 6
)
*/
print_r(getDescendants(0,$itemsByParent));
/*
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
[5] => 6
)
*/
Output of printList:
- Home
- News
- Sub News
- News
- Articles
- Article
- Article2
[ view entry ] ( 2347 views ) | print article
| 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | Next> Last>>